How to set properties of lines in a chart?
Code:
#Set Line Properties
from openpyxl import Workbook, load_workbook
from openpyxl.chart import LineChart, Reference
from openpyxl.chart.marker import DataPoint,Marker
from openpyxl.chart.shapes import GraphicalProperties
from openpyxl.drawing.colors import ColorChoice
from openpyxl.drawing.line import LineProperties
# Read the file
wb=load_workbook('Sales.xlsx')
# Select the first worksheet
ws=wb.worksheets[0]
# Get the data from the range B1:D6 and save it as a Reference object
data=Reference(ws, min_col=2, min_row=1, max_col=4, max_row=6)
# Get the data from the range A2:A6 for the x-axis of the bar chartlabels=Reference(ws, min_col=1, min_row=2, max_col=1, max_row=6)
labels=Reference(ws, min_col=1, min_row=2, max_col=1, max_row=6)
# Create a bar chart object
chart=LineChart()
# Set the data source
chart.add_data(data, titles_from_data=True)
# Set x-axis labels
chart.set_categories(labels)
#Set line properties for Series 1
chart.series[0].graphicalProperties.line=LineProperties(
solidFill='FF0000',
prstDash='sysDot',
w=50050
)
#Set line properties for Series 2
chart.series[1].graphicalProperties.line=LineProperties(
solidFill='00FF00',
prstDash='sysDash',
w=25050
)
#Set line properties for Series 3
chart.series[2].graphicalProperties.line=LineProperties(
solidFill='0000FF',
prstDash='solid',
w=20050
)
# Insert the chart into the worksheet
ws.add_chart(chart, 'E3')
# Save the workbook
wb.save('test.xlsx')
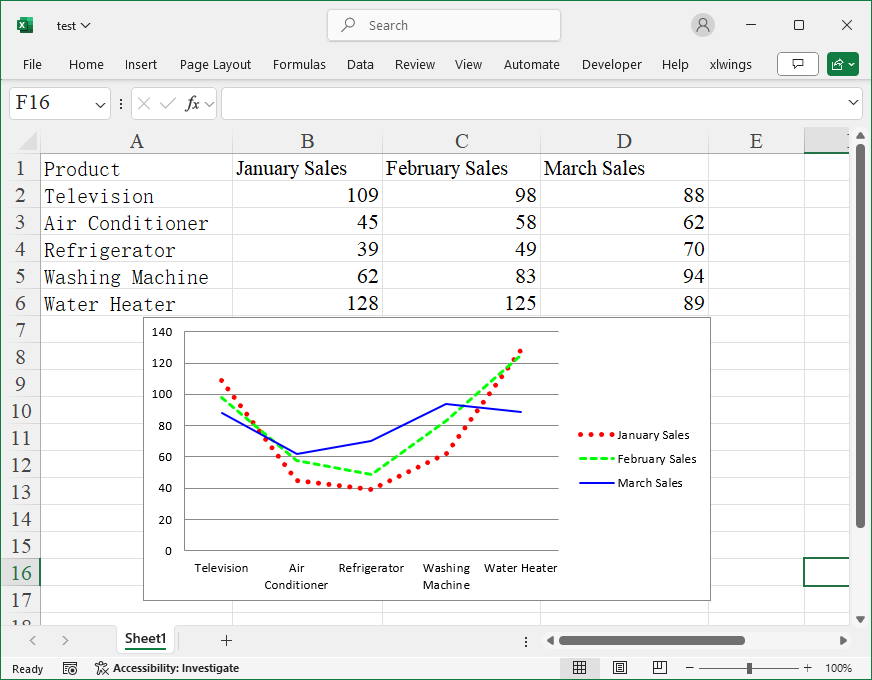